在 Uni-app 中自定义一个支持横向和竖向滑动的 Slider 滑块,并确保在页面旋转时能够正常使用,你需要结合 swiper
组件或者原生的触摸事件来实现。由于 swiper
组件主要用于轮播图或页面的滑动切换,而不是单个滑块的滑动,因此使用原生的触摸事件可能更为灵活。
以下是一个使用原生触摸事件实现的自定义 Slider 滑块的代码案例:
<template> | |
<viewclass=“container”> | |
<view | |
class=“slider-track” | |
:style=“{ width: trackWidth + ‘px’, height: trackHeight + ‘px’ }” | |
> | |
<view | |
class=“slider-thumb” | |
:style=“{ left: thumbLeft + ‘px’, top: thumbTop + ‘px’ }” | |
@touchstart=“onTouchStart” | |
@touchmove=“onTouchMove” | |
@touchend=“onTouchEnd” | |
></view> | |
</view> | |
<viewclass=“slider-value”>{{ currentValue }}</view> | |
</view> | |
</template> | |
<script> | |
exportdefault { | |
data() { | |
return { | |
containerWidth: 0, | |
containerHeight: 0, | |
trackWidth: 300, | |
trackHeight: 150, | |
thumbSize: 50, | |
thumbLeft: 0, | |
thumbTop: (150 – 50) / 2, // 初始时滑块在中间位置 | |
startX: 0, | |
startY: 0, | |
currentValue: 0, // 滑块当前的值,可以根据需要计算 | |
isDragging: false, | |
orientation: ‘horizontal’, // 初始方向为横向 | |
}; | |
}, | |
methods: { | |
onTouchStart(e) { | |
this.isDragging = true; | |
this.startX = e.touches[0].pageX; | |
this.startY = e.touches[0].pageY; | |
this.updateOrientation(); | |
}, | |
onTouchMove(e) { | |
if (!this.isDragging) return; | |
let deltaX = e.touches[0].pageX – this.startX; | |
let deltaY = e.touches[0].pageY – this.startY; | |
if (this.orientation === ‘horizontal’) { | |
let newLeft = this.thumbLeft + deltaX; | |
newLeft = Math.max(0, Math.min(this.trackWidth – this.thumbSize, newLeft)); | |
this.thumbLeft = newLeft; | |
this.currentValue = (newLeft / (this.trackWidth – this.thumbSize)) * 100; // 假设范围是0-100 | |
} else { | |
let newTop = this.thumbTop + deltaY; | |
newTop = Math.max(0, Math.min(this.trackHeight – this.thumbSize, newTop)); | |
this.thumbTop = newTop; | |
this.currentValue = (newTop / (this.trackHeight – this.thumbSize)) * 100; // 假设范围是0-100 | |
} | |
}, | |
onTouchEnd() { | |
this.isDragging = false; | |
}, | |
updateOrientation() { | |
// 根据窗口尺寸变化或其他条件动态判断方向 | |
// 这里简化处理,假设屏幕宽度大于高度时为横向,否则为竖向 | |
const windowWidth = uni.getSystemInfoSync().windowWidth; | |
const windowHeight = uni.getSystemInfoSync().windowHeight; | |
this.orientation = windowWidth > windowHeight ? ‘horizontal’ : ‘vertical’; | |
// 根据方向调整滑块初始位置和尺寸(如果需要) | |
// 例如,如果改为竖向,可能需要重新计算thumbTop等 | |
// 这里已经初始化时设置了thumbTop,所以竖向时不需要额外处理 | |
}, | |
}, | |
onLoad() { | |
// 页面加载时获取容器尺寸(如果需要动态计算) | |
// 这里简化处理,直接使用了固定的trackWidth和trackHeight | |
// 你可以通过uni.createSelectorQuery等方式获取实际尺寸 | |
}, | |
onShow() { | |
// 页面显示时更新方向(例如处理屏幕旋转) | |
this.updateOrientation(); | |
}, | |
}; | |
</script> | |
<stylescoped> | |
.container { | |
display: flex; | |
flex-direction: column; | |
align-items: center; | |
justify-content: center; | |
height: 100%; | |
} | |
.slider-track { | |
position: relative; | |
width: 100%; /* 可以根据需要调整 */ | |
max-width: 300px; /* 限制最大宽度 */ | |
height: 150px; /* 固定高度 */ | |
background-color: #ddd; | |
border-radius: 10px; | |
overflow: hidden; | |
} | |
.slider-thumb { | |
position: absolute; | |
width: 50px; | |
height: 50px; | |
background-color: #007aff; | |
border-radius: 50%; | |
transition: left 0.1s, top 0.1s; /* 添加过渡效果 */ | |
} | |
.slider-value { | |
margin-top: 20px; | |
font-size: 18px; | |
text-align: center; | |
} | |
</style> |
在这个示例中,slider-track
是滑块的轨道,slider-thumb
是滑块本身。通过监听触摸事件(touchstart
、touchmove
、touchend
),我们可以计算出滑块的位置,并更新其样式。同时,我们还添加了一个 updateOrientation
方法来根据屏幕方向动态调整滑块的方向。
请注意,这个示例中的 currentValue
是根据滑块位置计算出的一个百分比值(0-100)。你可以根据需要将其转换为其他形式的值,比如具体的数值范围。
此外,由于 Uni-app 支持多端发布,包括小程序、H5、App 等,因此在实际开发中,你可能需要根据不同平台的特性进行适配和测试。特别是在小程序中,触摸事件的处理可能会受到一些限制和性能影响。如果需要在小程序中使用,请确保测试其在各个平台上的表现。
一、了解《uni-app》与slider滑块
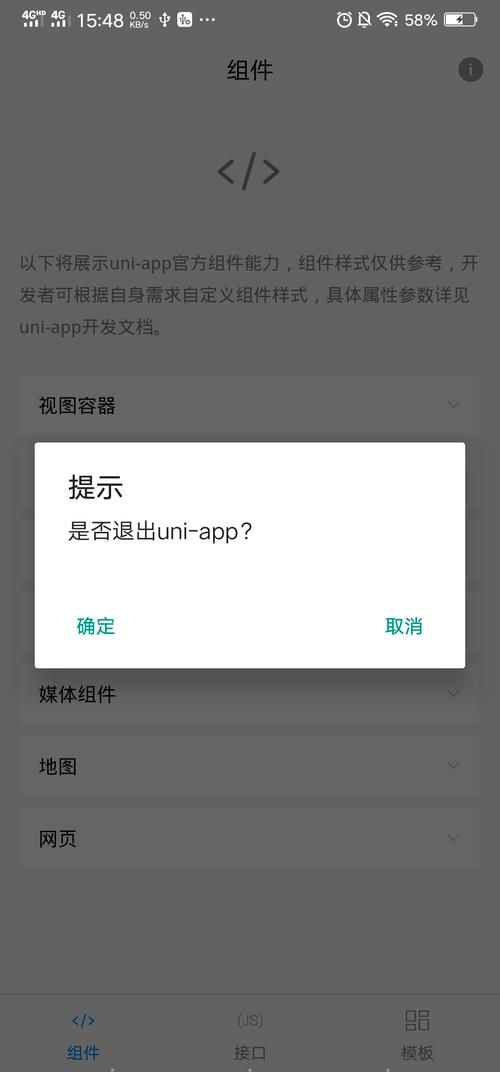
首先,你得知道,《uni-app》是一款使用vue.js开发所有前端应用的框架,它能够编译到iOS、Android、H5、以及各种小程序等多个平台。而slider滑块,则是一种常见的交互组件,可以让用户通过滑动来选择值或者切换内容。
二、创建自定义slider滑块
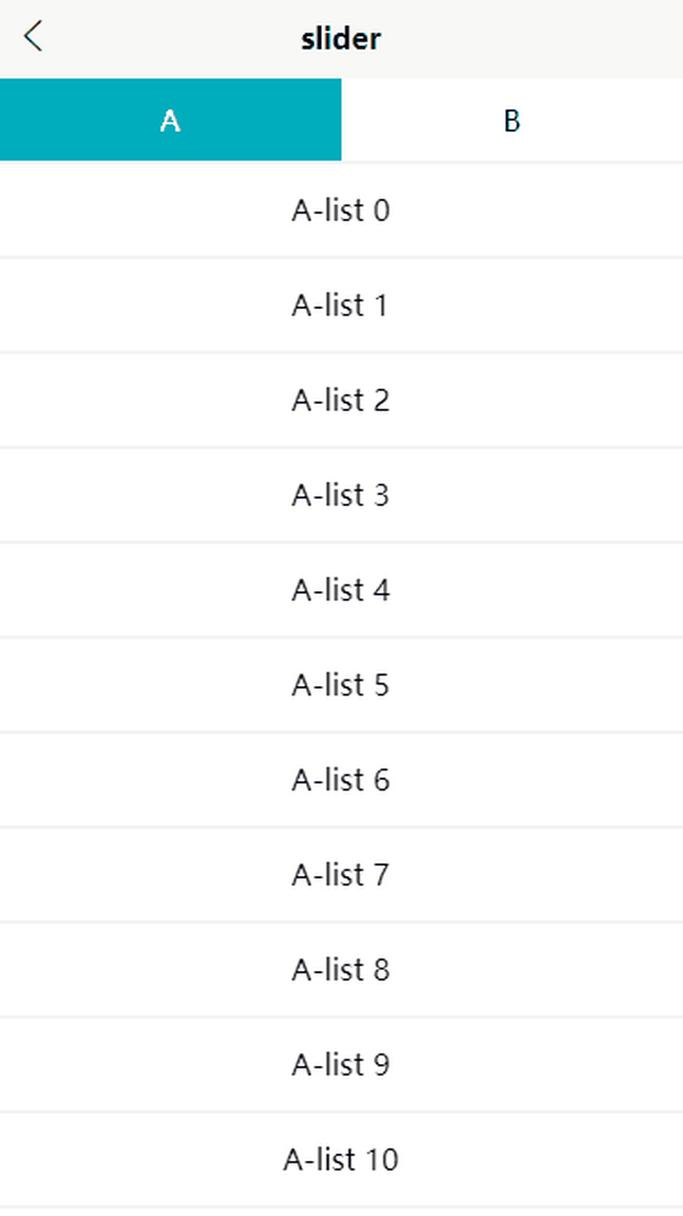
在这里,我们通过`handleChange`方法来更新滑块的值,通过`handleMoving`方法来监听滑块的移动。
三、实现竖向滑动
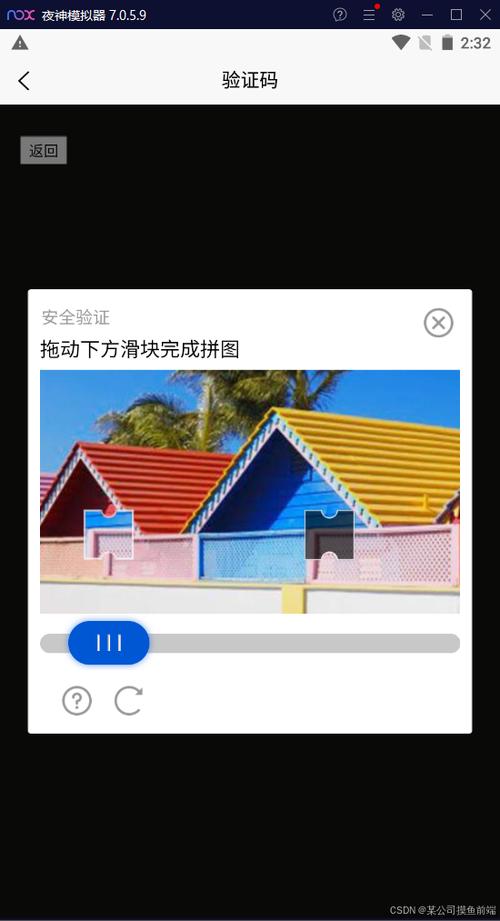
如果你想要实现竖向滑动,只需要将`direction`属性从`horizontal`改为`vertical`即可。其他部分的代码保持不变。
四、兼容页面旋转
为了确保滑块在页面旋转时仍然正常使用,我们需要在页面的`onReady`生命周期钩子中添加旋转监听:
“`javascript
export default {
onReady() {
uni.onDeviceOrientationChange((res) => {
if (res.value === 1) {
// 竖屏
this.direction = ‘vertical’;
} else {
// 横屏
this.direction = ‘horizontal’;
}
});
这样,无论用户如何旋转设备,滑块都会根据屏幕方向自动调整滑动方向。
在这个案例中,我们引入了自定义的`CustomSlider`组件,并设置了滑块的初始值、最小值、最大值和步长。
怎么样,是不是觉得这个自定义slider滑块特别酷呢?赶紧动手试试吧!相信通过你的创意和这个技巧,你的应用界面会更加生动有趣。加油哦!